About a decade ago, the JavaScript developer community began to witness fierce battles emerging among JavaScript frameworks. In this article, I will introduce some of the most well-known of these frameworks. And it's important to note that these are all open source JavaScript projects, meaning that you can freely utilize them under an open source license and even contribute to the source code and communities.
If you prefer to follow along as I explore these frameworks, you can watch my video.
Before getting started, though, it will be useful to learn some of the terminology JavaScript developers commonly use when discussing frameworks.
Term | What It Is |
---|---|
Document Object Model (DOM) | A tree-structure representation of a website where each node is an object representing part of the webpage. The World Wide Web Consortium (W3C), the international standards organization for the World Wide Web, maintains the definition of the DOM. |
Virtual DOM | A "virtual" or "ideal" representation of a user interface (UI) is kept in memory and synced with the "real" DOM by a library such as ReactDOM. To explore further, please read ReactJS's virtual DOM and internals documentation. |
Data Binding | A programming concept to provide a consistent interface for accessing data on websites. Web elements are associated with a property or attribute of an element maintained by the DOM. For example, when you need to fill out a password in a webpage form, the data binding mechanism can check with the password validation logic to ensure that the password is in a valid format. |
Now that we are clear about common terms, let's explore what open source JavaScript frameworks are out there.
Framework | About | License | Release Date |
---|---|---|---|
ReactJS | Created by Facebook, currently the most popular JavaScript framework | MIT License | May 24, 2013 |
Angular | Popular JavaScript framework created by Google | MIT License | Jan 5, 2010 |
VueJS | Rapidly growing JavaScript framework | MIT License | Jul 28, 2013 |
MeteorJS | Powerful framework that is more than a JavaScript framework | MIT License | Jan 18, 2012 |
KnockoutJS | Open source Model-View-ViewModel (MVVM) framework | MIT License | Jul 5, 2010 |
EmberJS | Another open source Model-View-ViewModel framework | MIT License | Dec 8, 2011 |
BackboneJS | JavaScript framework with RESTful JSON and Model-View-Presenter pattern | MIT License | Sep 30, 2010 |
Svelte | Open source JavaScript framework not using virtual DOM | MIT License | Nov 20, 2016 |
AureliaJS | A collection of Modern JavaScript modules | MIT License | Feb 14, 2018 |
For context, here is the publicly available data on popularity based on NPM package downloads per framework, thanks to npm trends.
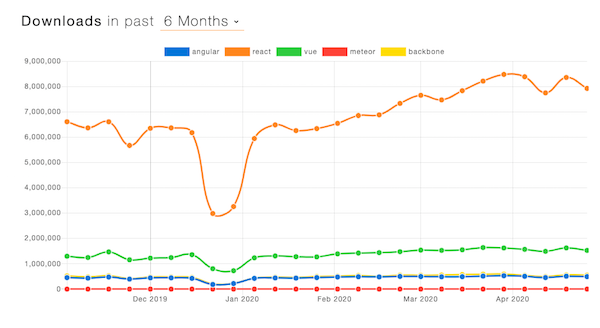
ReactJS
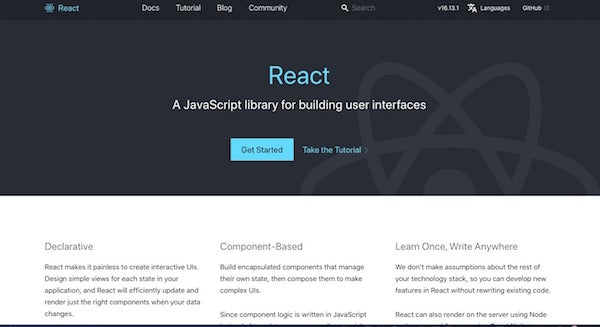
ReactJS was invented by Facebook, and it is the clear leader among JavaScript frameworks today, though it was invented well after Angular. React introduces a concept called a virtual DOM, an abstract copy where developers can utilize only the ReactJS features that they want, without having to rewrite the entire project to work within the framework. In addition, the active open source community with the React project has definitely been the workhorse behind the growth. Here are some of React's key strengths:
- Reasonable learning curve—React developers can easily create the React components without rewriting the entire code in JavaScript. See the benefits of ReactJS and how it makes it the programming easier on ReactJS's front page.
- Highly optimized for performance—React's virtual DOM implementation and other features boost app rendering performance. See ReactJS's description of how its performance can be benchmarked and measured in terms of how the app performs.
- Excellent supporting tools—Redux, Thunk, and Reselect are some of the best tools for building well-structured, debuggable code.
- One way data binding—The model used in Reach flows from owner to child only making it simpler to trace cause and effect in code. Read more about it on ReactJS's page on data binding.
Who is using ReactJS? Since Facebook invented it, the company itself heavily uses React for its frontpage, and Instagram is said to be completely based on the ReactJS library. You might be surprised to know that other well-known companies like New York Times, Netflix, and Khan Academy also implement ReactJS in their technology stacks.
What may be even more surprising is the availability of jobs for ReactJS developers, as you can see below from research done by Stackoverflow. Hey, you can work on an open source project and get paid to do it. That is pretty cool!
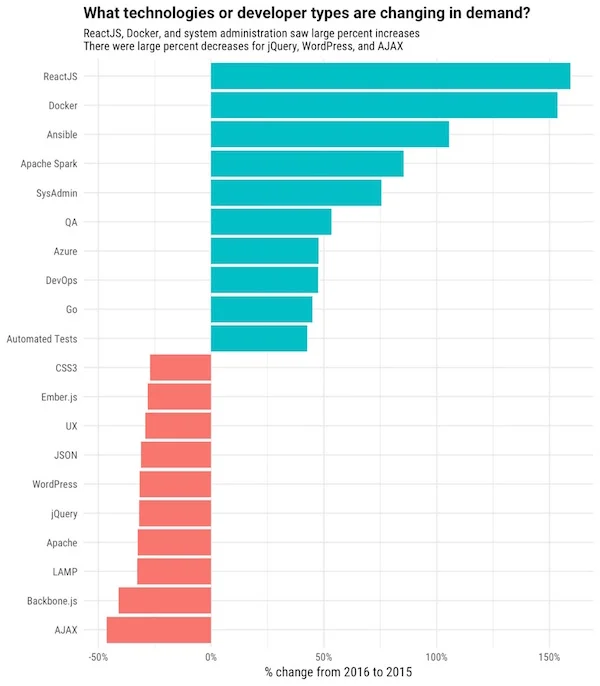
Stackoverflow shows the huge demand for ReactJS developers—Source: Developer Hiring Trends in 2017 - Stackoverflow Blog
ReactJS's GitHub currently shows over 13K commits and 1,377 contributors to the open source project. And it is an open source project under MIT License.
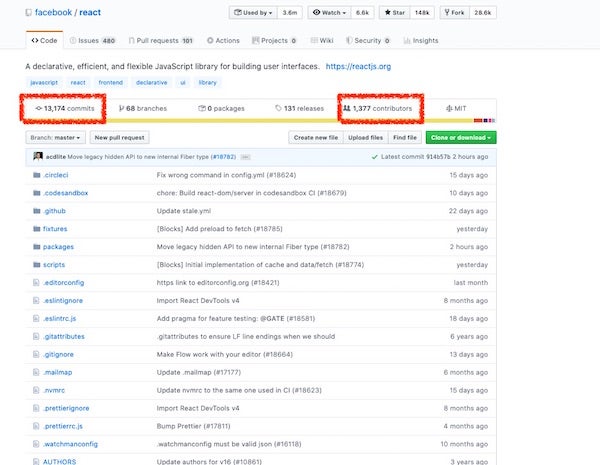
Angular
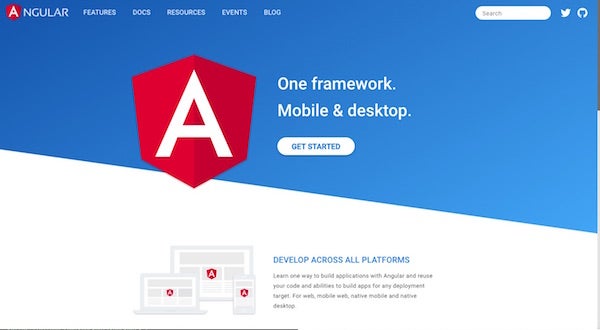
React may now be the leading JavaScript framework in terms of the number of developers, but Angular is close behind. In fact, while React is the more popular choice among open source developers and startup companies, larger corporations tend to prefer Angular (a few are listed below). The main reason is that, while Angular might be more complicated, its uniformity and consistency works well for larger projects. For example, I have worked on both Angular and React throughout my career, and I observed that the larger companies generally consider Angular's strict structure a strength. Here are some other key strengths of Angular:
- Well-designed Command Line Interface: Angular has an excellent Command Line Interface (CLI) tool to easily bootstrap and to develop with Angular. ReactJS also offers the Command Line Interface as well as other tools, but Angular has extensive support and excellent documentation, as you can see on this page.
- One way data binding—Similarly to React, the one-way data binding model makes the framework less susceptible to unwanted side effects.
- Great support for TypeScript—Angular has excellent alignment with TypeScript, which is effectively JavaScript more type enforcement. It also transcompiling to JavaScript, making it a great option to enforce types for less buggy code.
Well-known websites like YouTube, Netflix, IBM, and Walmart all have implemented Angular into their applications. I personally started front-end JavaScript development with Angular by educating myself on the subject. I have worked on quite a few personal and professional projects involving Angular, but that was Angular 1.x, which was called by AngularJS at the time. When Google decided to upgrade the version to 2.0, they completely revamped the framework, and then it became Angular. The new Angular was a complete transformation of the previous AngularJS, which drove off some existing developers while bringing new developers.
Angular's GitHub page shows 17,781 commits and 1,133 contributors at the time of this writing. It is also an open source project with an MIT License, so you can feel free to use it for your project or to contribute.
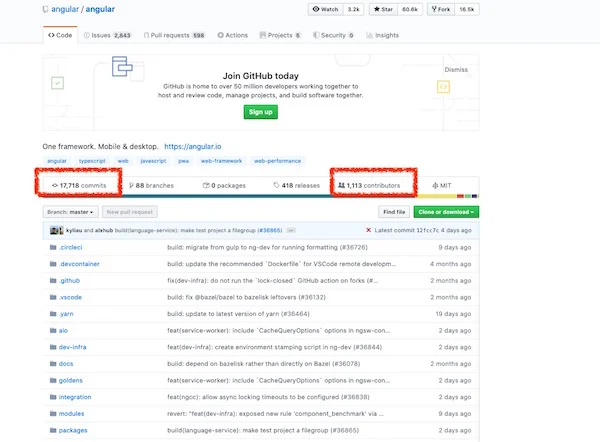
VueJS
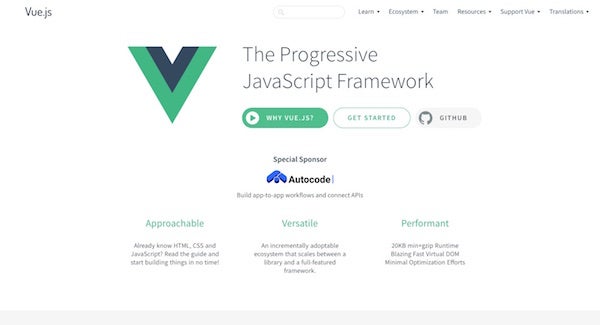
VueJS is a very interesting framework. It is a newcomer to the JavaScript framework scene, but its popularity has increased significantly over the course of a few years. VueJS was created by Evan Yu, a former Google engineer who had worked on the Angular project. The framework got so popular that many front-end engineers now prefer VueJS over other JavaScript frameworks. The chart below depicts how fast the framework gained traction over time.
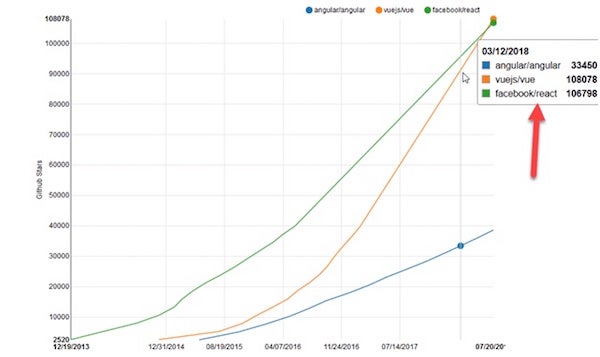
Here are some of the key strengths of VueJS:
- Easier learning curve—Even compared to Angular or React, many front-end developers feel that VueJS has the lowest learning curve.
- Small size—VueJS is relatively lightweight compared to Angular or React. In its official documentation, its size is stated to be only about 30 KB, while the project generated by Angular, for example, is over 65 KB.
- Concise documentation—Vue has thorough but concise and clear documentation. See its official documentation for yourself.
VueJS's GitHub shows 3,099 commits and 239 contributors.
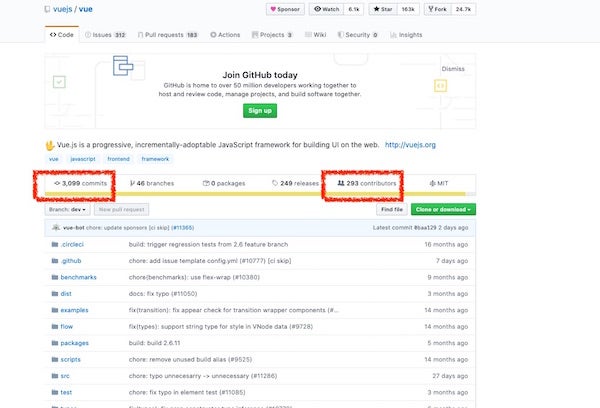
MeteorJS
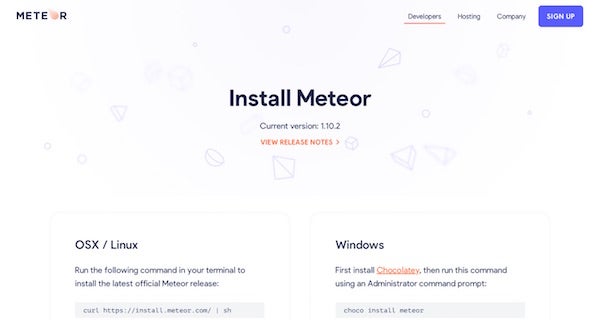
MeteorJS is a free and open source isomorphic framework, which means, just like NodeJS, it runs both client and server-side JavaScript. Meteor can be used in conjunction with any other popular front-end framework like Angular, React, Vue, Svelte, etc.
Meteor is used by several corporations such as Qualcomm, Mazda, and Ikea, and many applications like Dispatch and Rocket.Chat. See the case studies on its official website.
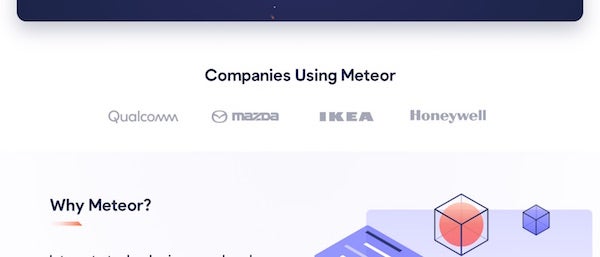
Some of the key features of Meteor include:
- Data on the wire—The server sends the data, not HTML, and the client renders it. Data on the wire refers mostly to the way that Meteor forms a WebSocket connection to the server on page load, and then transfers the data needed over that connection.
- Develop everything in JavaScript—Client-side, application server, webpage, and mobile interface can be all designed with JavaScript.
- Supports most major frameworks—Angular, React, and Vue can be all combined and used in conjunction with Meteor. Thus, you can still use your favorite framework, like React or Angular, but still leverage some of the great features that Meteor offers.
As of now, Meteor's GitHub shows 22,804 commits and 428 contributors. That is quite a lot for open source activities!
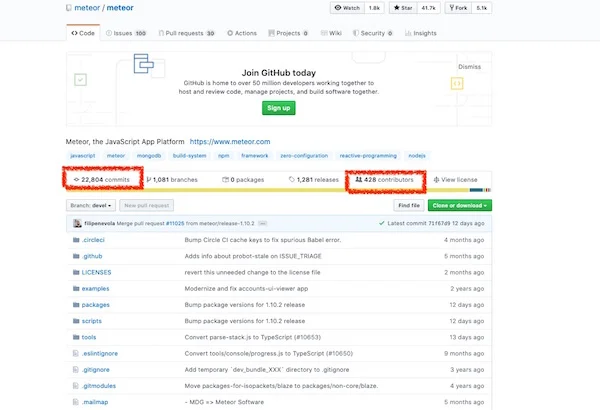
EmberJS
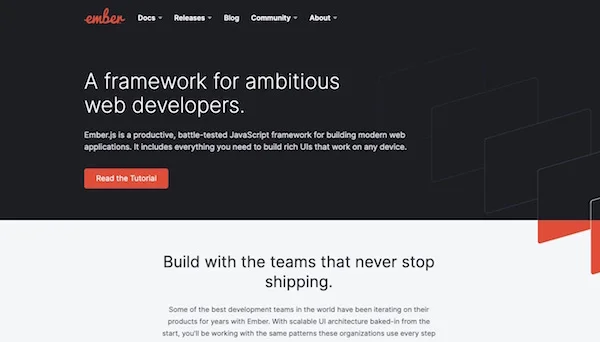
EmberJS is an open source JavaScript framework based on the Model-view-viewModel(MVVM) pattern. If you've never heard about EmberJS, you'll definitely be surprised how many companies are using it. Apple Music, Square, Discourse, Groupon, LinkedIn, Twitch, Nordstorm, and Chipotle all leverage EmberJS as one of their technology stacks. Check EmberJS's official page to discover all applications and customers that use EmberJS.
Although Ember has similar benefits to the other frameworks we've discussed, here are some of its unique differentiators:
- Convention over configuration—Ember standardizes naming conventions and automatically generates the result code. This approach has a little more of a learning curve but ensures that programmers follow best-recommended practices.
- Fully-fledged templating mechanism—Ember relies on straight text manipulation, building the HTML document dynamically while knowing nothing about DOM.
As one might expect from a framework used by many applications, Ember's GitHub page shows 19,808 commits and 785 contributors. That is huge!
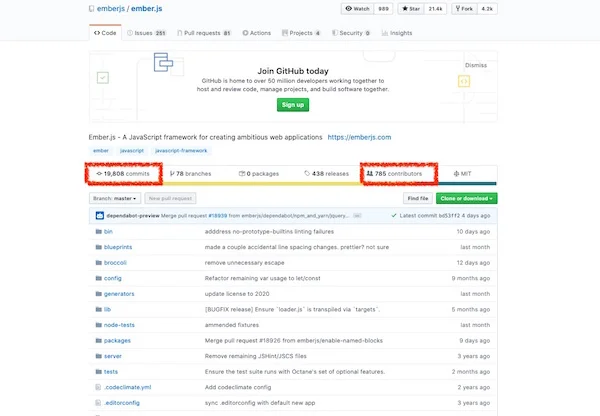
KnockoutJS
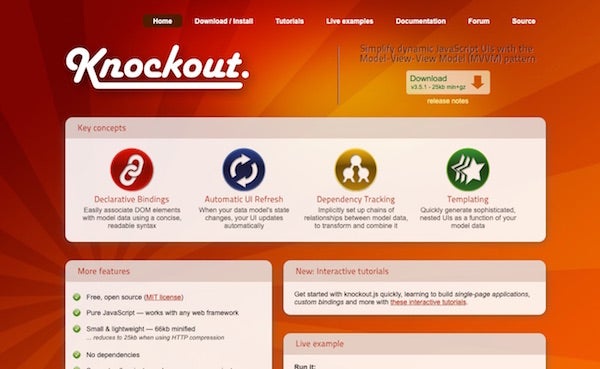
KnockoutJS is a standalone open source JavaScript framework adopting a Model-View-ViewModel (MVVM) pattern with templates. Although fewer people may have heard about this framework compared to Angular, React, or Vue, the project is still quite active among the development community and leverages features such as:
- Declarative binding—Knockout's declarative binding system provides a concise and powerful way to link data to the UI. It's generally easy to bind to simple data properties or to use a single binding. Read more about it here at KnockoutJS's official documentation page.
- Automatic UI refresh
- Dependency tracking templating
Knockout's GitHub page shows about 1,766 commits and 81 contributors. Those numbers are not as significant compared to the other frameworks, but the project is still actively maintained.
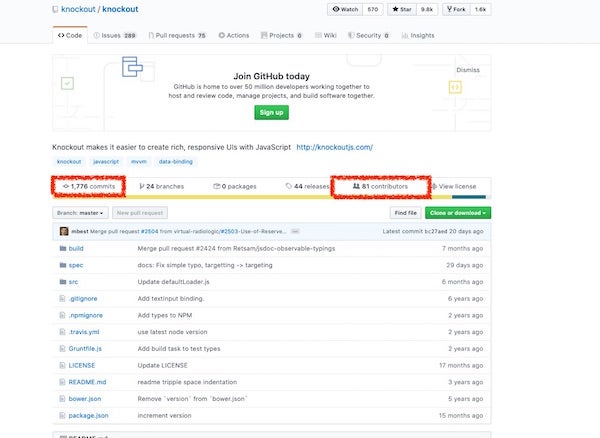
BackboneJS
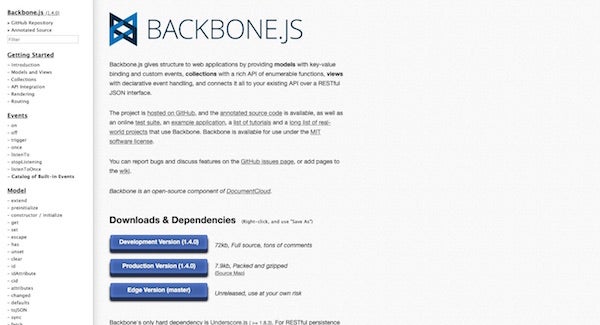
BackboneJS is a lightweight JavaScript framework with a RESTful JSON interface and is based on Model-View-Presenter (MVP) design paradigm.
The framework is said to be used by Airbnb, Hulu, SoundCloud, and Trello. You can find all of these case studies on Backbone's page.
The BackboneJS GitHub page shows 3,386 commits and 289 contributors.
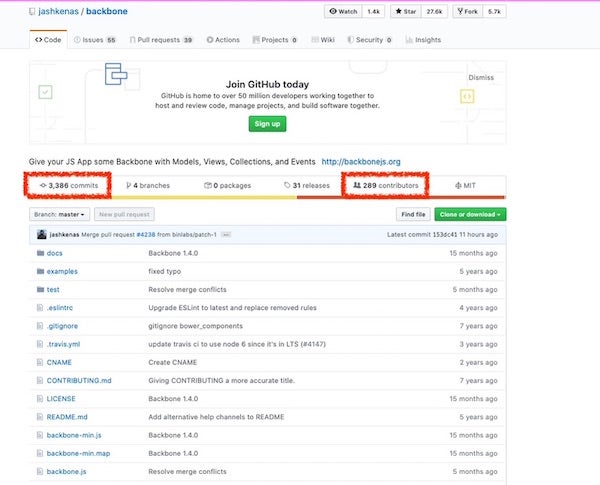
Svelte
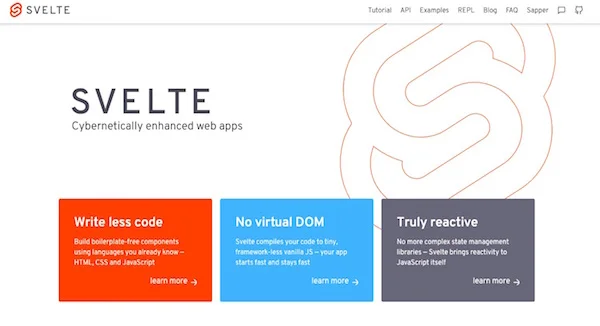
Svelte is an open source JavaScript framework that generates the code to manipulate DOM instead of including framework references. This process of converting an app into JavaScript at build time rather than run time might offer a slight boost in performance in some scenarios.
Svelte's GitHub page shows 5,729 commits and 296 contributors as of this writing.
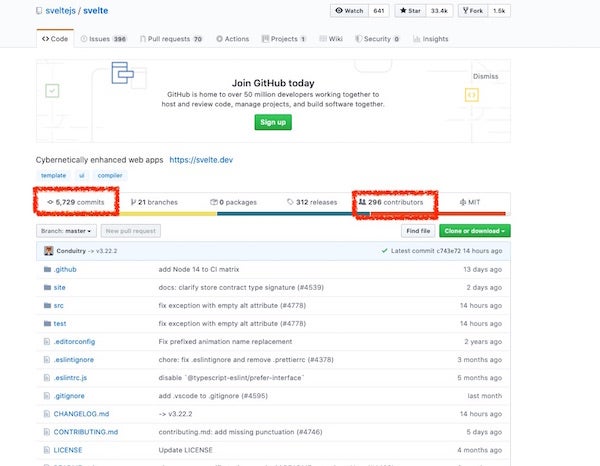
AureliaJS
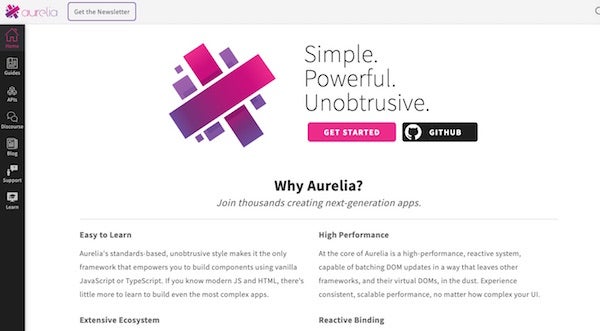
Last on the list is Aurelia. Aurelia is a front-end JavaScript framework that is a collection of modern JavaScript modules. Aurelia has the following interesting features:
- Fast rendering—Aurelia claims that its framework can render faster than any other framework today.
- Uni-directional data flow—Aurelia uses an observable-based binding system that pushes the data from the model to the view.
- Build with vanilla JavaScript—You can build all of the website's components with plain JavaScript.
Aurelia's GitHub page shows 788 commits and 96 contributors as of this writing.
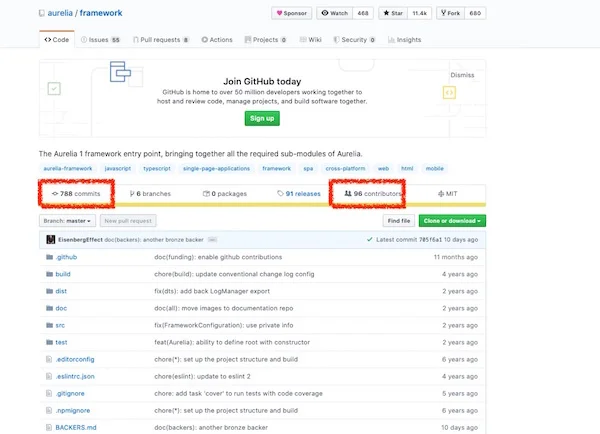
So that is what I found when looking at what is new in the JavaScript framework world. Did I miss any interesting frameworks? Feel free to share your ideas in the comment section!
6 Comments