Django developers, we're devoting this month's Python column to packages that will help you. These are our favorite Django libraries for saving time, cutting down on boilerplate code, and generally simplifying our lives. We've got six packages for Django apps and two for Django's REST Framework, and we're not kidding when we say these packages show up in almost every project we work on.
But first, see our tips for making the Django Admin more secure and an article on 5 favorite open source Django packages.
A kitchen sink of useful time-savers: django-extensions
Django-extensions is a favorite Django package chock full of helpful tools like these management commands:
- shell_plus starts the Django shell with all your database models already loaded. No more importing from several different apps to test one complex relationship!
- clean_pyc removes all .pyc projects from everywhere inside your project directory.
- create_template_tags creates a template tag directory structure inside the app you specify.
- describe_form displays a form definition for a model, which you can then copy/paste into forms.py. (Note that this produces a regular Django form, not a ModelForm.)
- notes displays all comments with stuff like TODO, FIXME, etc. throughout your project.
Django-extensions also includes useful abstract base classes to use for common patterns in your own models. Inherit from these base classes when you create your models to get their:
- TimeStampedModel: This base class includes the fields created and modified and a save() method that automatically updates these fields appropriately.
- ActivatorModel: If your model will need fields like status, activate_date, and deactivate_date, use this base class. It comes with a manager that enables .active() and .inactive() querysets.
- TitleDescriptionModel and TitleSlugDescriptionModel: These include the title and description fields, and the latter also includes a slug field. The slug field will automatically populate based on the title field.
Django-extensions has more features you may find useful in your projects, so take a tour through its docs!
12-factor-app settings: django-environ
Django-environ allows you to use 12-factor app methodology to manage your settings in your Django project. It collects other libraries, including envparse and honcho. Once you install django-environ, create an .env file at your project's root. Define in that module any settings variables that may change between environments or should remain secret (like API keys, debug status, and database URLs).
Then, in your project's settings.py file, import environ and set up variables for environ.PATH() and environ.Env() according to the example. Access settings variables defined in your .env file with env('VARIABLE_NAME').
Creating great management commands: django-click
Django-click, based on Click (which we have recommended before… twice), helps you write Django management commands. This library doesn't have extensive documentation, but it does have a directory of test commands in its repository that are pretty useful. A basic Hello World command would look like this:
# app_name.management.commands.hello.py
import djclick as click
@click.command()
@click.argument('name')
def command(name):
click.secho(f'Hello, {name}')
Then in the command line, run:
>> ./manage.py hello Lacey
Hello, Lacey
Handling finite state machines: django-fsm
Django-fsm adds support for finite state machines to your Django models. If you run a news website and need articles to process through states like Writing, Editing, and Published, django-fsm can help you define those states and manage the rules and restrictions around moving from one state to another.
Django-fsm provides an FSMField to use for the model attribute that defines the model instance's state. Then you can use django-fsm's @transition decorator to define methods that move the model instance from one state to another and handle any side effects from that transition.
Although django-fsm is light on documentation, Workflows (States) in Django is a gist that serves as an excellent introduction to both finite state machines and django-fsm.
Contact forms: #django-contact-form
A contact form is such a standard thing on a website. But don't write all that boilerplate code yourself—set yours up in minutes with django-contact-form. It comes with an optional spam-filtering contact form class (and a regular, non-filtering class) and a ContactFormView base class with methods you can override or customize, and it walks you through the templates you will need to create to make your form work.
Registering and authenticating users: django-allauth
Django-allauth is an app that provides views, forms, and URLs for registering users, logging them in and out, resetting their passwords, and authenticating users with outside sites like GitHub or Twitter. It supports email-as-username authentication and is extensively documented. It can be a little confusing to set up the first time you use it; follow the installation instructions carefully and read closely when you customize your settings to make sure you're using all the settings you need to enable a specific feature.
Handling user authentication with Django REST Framework: django-rest-auth
If your Django development includes writing APIs, you're probably using Django REST Framework (DRF). If you're using DRF, you should check out django-rest-auth, a package that enables endpoints for user registration, login/logout, password reset, and social media authentication (by adding django-allauth, which works well with django-rest-auth).
Visualizing a Django REST Framework API: django-rest-swagger
Django REST Swagger provides a feature-rich user interface for interacting with your Django REST Framework API. Once you've installed Django REST Swagger and added it to installed apps, add the Swagger view and URL pattern to your urls.py file; the rest is taken care of in the docstrings of your APIs.
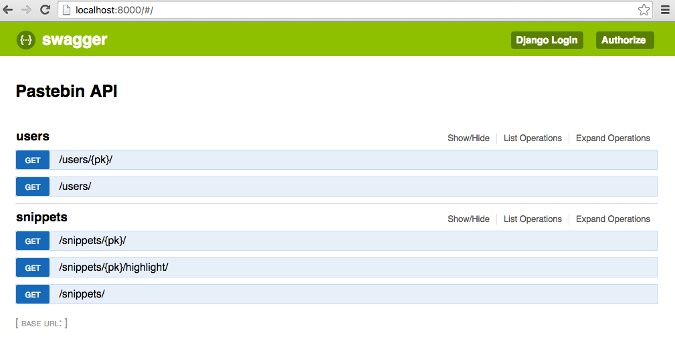
The UI for your API will include all your endpoints and available methods broken out by app. It will also list available operations for those endpoints and enable you to interact with the API (adding/deleting/fetching records, for example). It uses the docstrings in your API views to generate documentation for each endpoint, creating a set of API documentation for your project that's useful to you, your frontend developers, and your users.
Comments are closed.