What's not to love about Java?
I love that Java lets me write applications on one platform and run them on other platforms. You don't have to mess around with platform-specific SDKs, using a different library for that one platform, or inserting little code hacks to make that other platform behave. To me, that's how easy all modern programming ought to be. There's great infrastructure around Java, too, like the Maven build system and SDKMan.
But there's one thing about Java I don't love: the look and feel of its default GUI toolkit, called Swing. While some people feel there's a charming nostalgia to Swing, for the modern computerist, it can look a little dated.
Fortunately, that's an easy problem to solve. Java is a programming language, so if you're writing an app in Java you can reprogram its look and feel using one of several Java theming libraries.
Default theme of Java Swing
The default theme of Java Swing hasn't changed much in the past decade. This is a screenshot of an example Java app from 2006—or is it 2022?
Hard to tell.
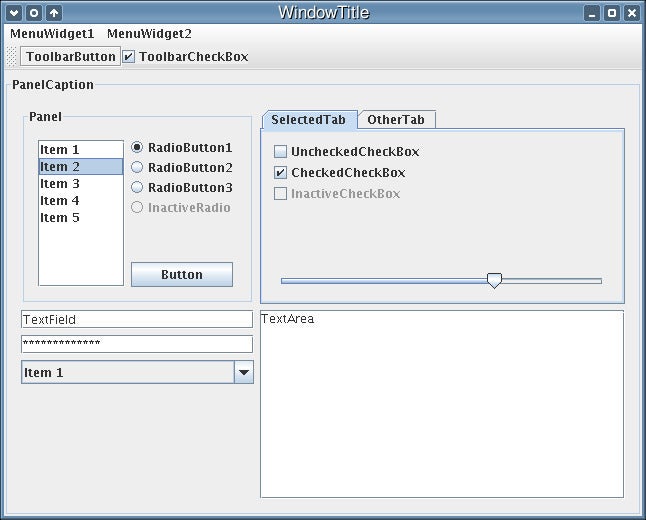
(Faxe, GPLv3)
You can see a simpler version for yourself with this demo code:
package com.opensource.myexample2app;
import javax.swing.Box;
import javax.swing.BoxLayout;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.border.EmptyBorder;
import java.awt.Dimension;
import java.awt.EventQueue;
import java.awt.Insets;
import javax.swing.JRadioButton;
public class App extends JFrame {
private void run() {
var window = new JPanel();
window.setLayout(new BoxLayout(window, BoxLayout.X_AXIS));
window.setBorder(new EmptyBorder(new Insets(15, 15, 15, 15)));
JButton btn_hello = new JButton("Hello");
btn_hello.setSize(new Dimension(80, 20));
window.add(btn_hello);
window.add(Box.createRigidArea(new Dimension(10, 15)));
JRadioButton rad_world = new JRadioButton("World");
rad_world.setSize(new Dimension(80, 20));
window.add(rad_world);
add(window);
pack();
setTitle("Hello world");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLocationRelativeTo(null);
}
public static void main(String[] args) {
EventQueue.invokeLater(() -> {
var app = new App();
app.run();
app.setVisible(true);
});
}
}
Run that in the Java IDE of your choice or with Java directly:
$ java App.java
The result is simple but demonstrative:
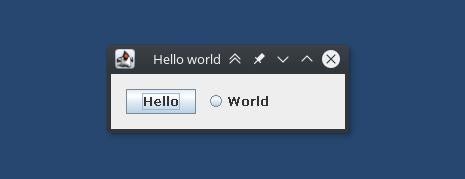
(Seth Kenlon, CC BY-SA 4.0)
Look and Feel Java libraries
Java Swing gets its theme from Look and Feel (LAF) libraries.
The few that are bundled with Java suffice in a pinch, but newer ones are available, and you can bundle those libraries with your code to give your application a different theme. You can find LAF libraries by searching for "laf" on sites like Mvnrepository.com, or on popular coding sites like GitLab or GitHub.
My favorite is FlatLaf, but there are very good themes by NetBeans, IntelliJ, and many others under a variety of different licenses to fit any project.
The easiest way to use one of these libraries is to add it to Maven, then make minor modifications to your code to invoke the theme.
First, add the library to Maven:
<dependencies>
<dependency>
<groupId>com.formdev</groupId>
<artifactId>flatlaf</artifactId>
<version>2.0.1</version>
</dependency>
</dependencies>
Next, import the Java UIManager
library and the LAF theme you're using to your project.
import com.formdev.flatlaf.FlatLightLaf;
import javax.swing.UIManager;
In the class that creates your GUI, use UIManager
to set the application's look and feel:
try {
UIManager.setLookAndFeel( new FlatLightLaf() );
} catch( Exception ex ) {
System.err.println( "Failed to initialize theme. Using fallback." );
}
That's it! Launch your application to see the new look and feel.
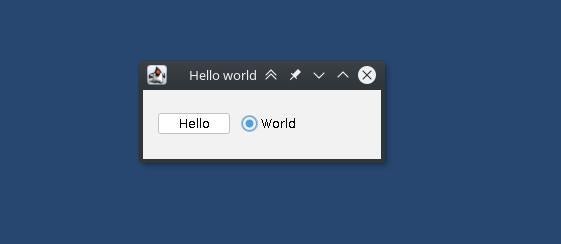
(Seth Kenlon, CC BY-SA 4.0)
Flatlaf happens to have variant themes, so you can change FlatLightLaf
to FlatDarkLaf
for a dark theme:
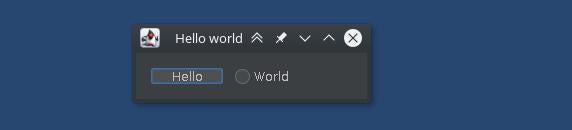
(Seth Kenlon, CC BY-SA 4.0)
Or use FlatIntelliJLaf
for an IntelliJ-like look and feel:
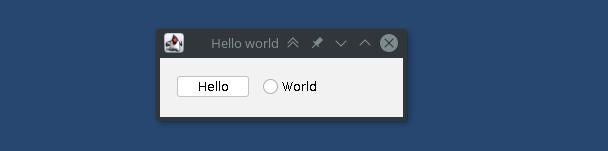
(Seth Kenlon, CC BY-SA 4.0)
Or FlatDarculaLaf
for a dark IntelliJ look and feel:
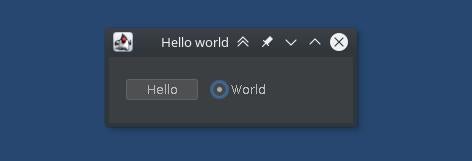
(Seth Kenlon, CC BY-SA 4.0)
Looking good, Java
Java Swing is an easy toolkit to use. It's been well maintained for two decades, and it provides a great desktop experience.
With look and feel libraries, Swing can look good, too.
Comments are closed.