In C++, reading and writing to files can be done by using I/O streams in conjunction with the stream operators >>
and <<
. When reading or writing to files, those operators are applied to an instance of a class representing a file on the hard drive. This stream-based approach has a huge advantage: From a C ++ perspective, it doesn't matter what you are reading or writing to, whether it's a file, a database, the console, or another PC you are connected to over the network. Therefore, knowing how to write files using stream operators can be transferred to other areas.
I/O stream classes
The C++ standard library provides the class ios_base. This class acts as the base class for all I/O stream-compatible classes, such as basic_ofstream and basic_ifstream. This example will use the specialized types for reading/writing characters, ifstream
and ofstream
.
ofstream
means output file stream, and it can be accessed with the insertion operator,<<
.ifstream
means input file stream, and it can be accessed with the extraction operator,>>
.
Both types are defined inside the header <fstream>
.
A class that inherits from ios_base
can be thought of as a data sink when writing to it or as a data source when reading from it, completely detached from the data itself. This object-oriented approach makes concepts such as separation of concerns and dependency injection easy to implement.
A simple example
This example program is quite simple: It creates an ofstream
, writes to it, creates an ifstream
, and reads from it:
#include <iostream> // cout, cin, cerr etc...
#include <fstream> // ifstream, ofstream
#include <string>
int main()
{
std::string sFilename = "MyFile.txt";
/******************************************
* *
* WRITING *
* *
******************************************/
std::ofstream fileSink(sFilename); // Creates an output file stream
if (!fileSink) {
std::cerr << "Canot open " << sFilename << std::endl;
exit(-1);
}
/* std::endl will automatically append the correct EOL */
fileSink << "Hello Open Source World!" << std::endl;
/******************************************
* *
* READING *
* *
******************************************/
std::ifstream fileSource(sFilename); // Creates an input file stream
if (!fileSource) {
std::cerr << "Canot open " << sFilename << std::endl;
exit(-1);
}
else {
// Intermediate buffer
std::string buffer;
// By default, the >> operator reads word by workd (till whitespace)
while (fileSource >> buffer)
{
std::cout << buffer << std::endl;
}
}
exit(0);
}
This code is available on GitHub. When you compile and execute it, you should get the following output:
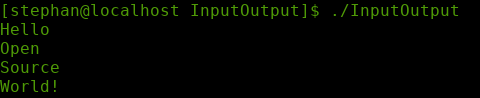
(Stephan Avenwedde, CC BY-SA 4.0)
This is a simplified, beginner-friendly example. If you want to use this code in your own application, please note the following:
- The file streams are automatically closed at the end of the program. If you want to proceed with the execution, you should close them manually by calling the
close()
method. - These file stream classes inherit (over several levels) from basic_ios, which overloads the
!
operator. This lets you implement a simple check if you can access the stream. On cppreference.com, you can find an overview of when this check will (and won't) succeed, and you can implement further error handling. - By default,
ifstream
stops at white space and skips it. To read line by line until you reach EOF, use thegetline(...)
-method. - For reading and writing binary files, pass the
std::ios::binary
flag to the constructor: This prevents EOL characters from being appended to each line.
Writing from the systems perspective
When writing files, the data is written to the system's in-memory write buffer. When the system receives the system call sync, this buffer's contents are written to the hard drive. This mechanism is also the reason you shouldn't remove a USB stick without telling the system. Usually, sync is called on a regular basis by a daemon. If you really want to be on the safe side, you can also call sync manually:
#include <unistd.h> // needs to be included
sync();
Summary
Reading and writing to files in C++ is not that complicated. Moreover, if you know how to deal with I/O streams, you also know (in principle) how to deal with any kind of I/O device. Libraries for various kinds of I/O devices let you use stream operators for easy access. This is why it is beneficial to know how I/O steams work.
Comments are closed.