Open source software projects often have a very diverse user group. Some users might be very adept at using the system and need very little documentation. For these power users, documentation might only need to be reminders and hints, and can include more technical information such as commands to run at the shell. But other users may be beginners. These users need more help in setting up the system and learning how to use it.
Writing documentation that suits both user groups can be daunting. The website's documentation needs to somehow balance "detailed technical information" with "providing more overview and guidance." This is a difficult balance to find. If your documentation can't meet both user groups, consider a third option — dynamic documentation.
Explore how to add a little JavaScript to a web page so the user can choose to display just the information they want to see.
Structure your content
You can use the example where documentation needs to suit both expert and novice users. In the simplest case, you can use a made-up music player called AwesomeProject.
You can write a short installation document in HTML that provides instructions for both experts and novices by using the class feature in HTML. For example, you can define a paragraph intended for experts by using:
<p class="expert reader">
This assigns both the expert class and the reader class. You can create a parallel set of instructions for novices using:
<p class="novice reader">
The complete HTML file includes both paragraphs for novice readers and experts:
<!DOCTYPE html>
<html lang="en">
<head>
<title>How to install the software</title>
</head>
<body>
<h1>How to install the software</h1>
<p>Thanks for installing AwesomeProject! With AwesomeProject,
you can manage your music collection like a wizard.</p>
<p>But first, we need to install it:</p>
<p class="expert reader">You can install AwesomeProject from
source. Download the tar file, extract it, then run:
<code>./configure ; make ; make install</code></p>
<p class="novice reader">AwesomeProject is available in
most Linux distributions. Check your graphical package manager and search for AwesomeProject to install it.</p>
</body>
</html>
This sample HTML document doesn't have a stylesheet associated with it, so viewing this in a web browser shows both paragraphs:
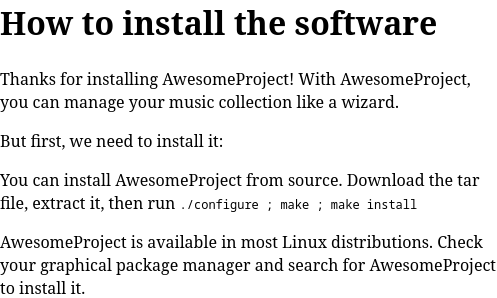
(Jim Hall, CC BY-SA 4.0)
We can apply some basic styling to the document to highlight any element with the reader, expert, or novice classes. To make the different text classes easier to differentiate, let's set the reader class to an off-white background color, expert to a dark red text color, and novice to a dark blue text color:
<!DOCTYPE html>
<html lang="en">
<head>
<title>How to install the software</title>
<style>
.reader {
background-color: ghostwhite;
}
.expert {
color: darkred;
}
.novice {
color: darkblue;
}
</style>
</head>
<body>
<h1>How to install the software</h1>
These styles help the two sections stand out when you view the page in a web browser. Both paragraphs with the installation instructions have an off-white background color because they both have the reader class. The first paragraph uses dark red text, as defined by the expert class. The second installation paragraph is in dark blue text, from the novice class:
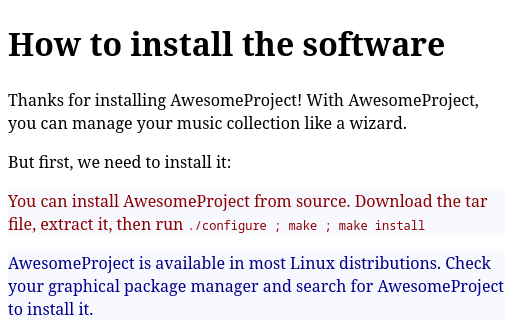
(Jim Hall, CC BY-SA 4.0)
Add JavaScript controls
With these classes applied, you can add a short JavaScript function that shows just one of the content blocks. One way to write this function is to first set display:none
to all of the elements with the reader class. This hides the content so it won't display on the page. Then the function should set display:block
to each of the elements with the class you want to display:
<script>
function readerview(audience) {
var list, item;
// hide all class="reader"
list = document.getElementsByClassName("reader");
for (item = 0; item < list.length; item++) {
list[item].style.display = "none";
}
// show all class=audience
list = document.getElementsByClassName(audience);
for (item = 0; item < list.length; item++) {
list[item].style.display = "block";
}
}
</script>
To use this JavaScript in the HTML document, you can attach the function to a button. Since the readerview
function takes an audience as its parameter, you can call the function with the audience class that you want to view, either novice or expert:
<!DOCTYPE html>
<html lang="en">
<head>
<title>How to install the software</title>
<style>
.reader {
background-color: ghostwhite;
}
.expert {
color: darkred;
}
.novice {
color: darkblue;
}
</style>
</head>
<body>
<script>
function readerview(audience) {
var list, item;
// hide all class="reader"
list = document.getElementsByClassName("reader");
for (item = 0; item < list.length; item++) {
list[item].style.display = "none";
}
// show all class=audience
list = document.getElementsByClassName(audience);
for (item = 0; item < list.length; item++) {
list[item].style.display = "block";
}
}
</script>
<h1>How to install the software</h1>
<nav>
<button onclick="readerview('novice')">view novice text</button>
<button onclick="readerview('expert')">view expert text</button>
</nav>
<p>Thanks for installing AwesomeProject! With AwesomeProject,
you can manage your music collection like a wizard.</p>
<p>But first, we need to install it:</p>
<p class="expert reader">You can install AwesomeProject from
source. Download the tar file, extract it, then run
<code>./configure ; make ; make install</code></p>
<p class="novice reader">AwesomeProject is available in
most Linux distributions. Check your graphical package
manager and search for AwesomeProject to install it.</p>
</body>
</html>
With these controls in place, the web page now allows the user to select the text they want to see:
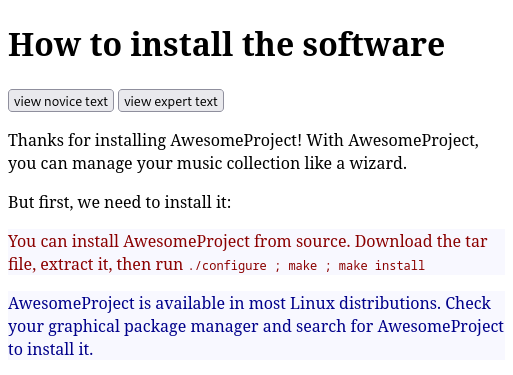
(Jim Hall, CC BY-SA 4.0)
Clicking either button will show just the text the user wants to read. For example, if you click the “view novice text” button, then you'll see just the blue paragraph:
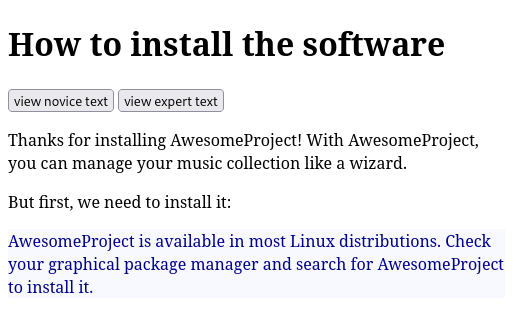
(Jim Hall, CC BY-SA 4.0)
Clicking the “view expert text” button hides the novice text and shows only the expert text in red:
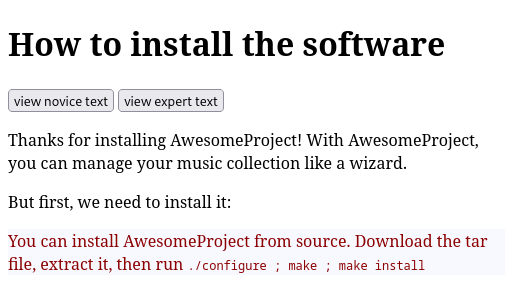
(Jim Hall, CC BY-SA 4.0)
Extend this to your documentation
If your project requires you to write multiple how-to documents for different audiences, consider using this method to publish once and read twice. Writing a single document for all your users makes it easy for everyone to find and share the documentation for your project. And you won't have to maintain parallel documentation that varies in just the details.
1 Comment