About a year ago, I took a course in Arabic. In addition to being a right-to-left written language, Arabic has its own alphabet. Since this was an introductory class, I spent most of my time working my way through the Arabic alphabet.
So I decided to create a study aid: It would present an Arabic letter, I would formulate a guess, and it would tell me whether or not I had answered correctly. Some brief experimentation, however, showed that this approach would not work—the letters appeared so small that I couldn’t be sure what I was seeing on the command line.
My next idea was to come up with some sort of window display of the glyph that was large enough to easily recognize. Since PyQt has these kinds of things built in, it seemed like the best approach to use.
Upon delving in, I discovered a number of resources on the web, and as I began to experiment, I needed to make sure all the code correlated. In particular, there have been changes in the organization of PyQt as it has gone from PyQt 4 to PyQt 5, and the latter requires Python 3.
After getting this all straightened out, here is what I came up with:
#!/usr/bin/python3
# -*- coding: utf-8 -*-
"""
arabic.py
An exercise for learning Arabic letters. An Arabic letter is shown, the name of which can the guessed.
Hover the mouse cursor over the letter to see its name in a Tool Tip. Click Next to load another
letter.
"""
import sys
from random import randint
from PyQt5.QtCore import *
from PyQt5.QtGui import QFont
from PyQt5.QtWidgets import QWidget, QLabel, QApplication, QToolTip, QPushButton
class Example(QWidget):
def __init__(self, parent = None):
super(Example, self).__init__(parent)
self.initUI()
def initUI(self):
QToolTip.setFont(QFont('DejaVuSans', 30))
i = randint(0, 37)
self.lbl2 = QLabel(L[12], self)
self.lbl2.setToolTip(lname[12]+' \n ')
self.lbl2.setFont(QFont('FreeSerif', 40))
self.lbl2.setTextFormat(1)
self.lbl2.setAlignment(Qt.AlignVCenter)
self.lbl2.setAlignment(Qt.AlignHCenter)
butn = QPushButton("Next", self)
butn.move(70, 70)
butn.clicked.connect(self.buttonClicked)
self.setGeometry(300, 300, 160, 110)
self.setWindowTitle('Arabic Letters')
self.show()
def buttonClicked(self):
i = randint(0,37)
self.lbl2.setFont(QFont('FreeSerif', 40))
self.lbl2.setTextFormat(1)
self.lbl2.setText(L[i])
self.lbl2.setAlignment(Qt.AlignVCenter)
self.lbl2.setAlignment(Qt.AlignHCenter)
self.lbl2.setToolTip(lname[i]+' \n ')
if __name__ == '__main__':
lname = ['alif','beh','teh','theh','jim','ha','kha','dal','dhal','ra','zin','sin','shin','Sad','Dad','Ta','DHa','ain','ghain','feh','qaf','kaf','lam','mim','nün','heh','waw','yeh', 'Sifr', 'wáaHid', 'ithnáyn', 'thaláatha','árba:ah', 'khámsah', 'sittah','sáb:ah', 'thamáanyah', 'tís:ah']
L = [u"\u0627", u"\u0628", u"\u062A", u"\u062B", u"\u062C",u"\u062D", u"\u062E", u"\u062F", u"\u0630", u"\u0631", u"\u0632", u"\u0633", u"\u0634", u"\u0635", u"\u0636", u"\u0637", u"\u0638", u"\u0639", u"\u063A", u"\u0641", u"\u0642", u"\u0643", u"\u0644", u"\u0645", u"\u0646", u"\u0647", u"\u0648", u"\u064A", u"\u0660", u"\u0661", u"\u0662", u"\u0663", u"\u0664", u"\u0665", u"\u0666", u"\u0667", u"\u0668", u"\u0669" ]
app = QApplication(sys.argv)
ex = Example()
sys.exit(app.exec_())
All of those import statements involving PyQt 5 are the result of much trial and error, but they cover everything I needed. I created a QWidget, which serves as the space to place the letters and contains a QButton labeled “Next” to proceed the next glyph. At the bottom you can see that I created two lists: The first, lname
, contains phonetic pronunciations of each letter and Arabic numeral. The second, L
, contains the Unicode value for each glyph.
At the top are the functions that set up the QWidget. It chooses a glyph to display and randomly selects a new one when you click the Next button. The pronunciations come in as a tooltip when you hover the mouse cursor over the glyph. You might notice that the first glyph is not randomly chosen; it’s L[12]
. This is a workaround for the fact that this first glyph determines the maximum display space for all subsequent glyphs, so I chose the widest glyph I could find to prevent subsequent glyphs from being chopped off.
Here is the widget, without and with the tooltip showing:
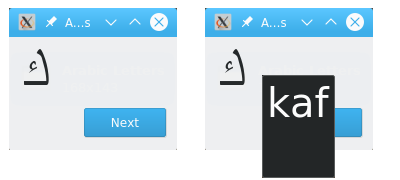
I found this tool very helpful in learning the Arabic glyphs. It could easily be adapted to other topics by simply changing the content of lname[]
and L[]
.
8 Comments